Browser script examples#
The following sections showcase ready-to-use browser scripts. See Using the Zyte IDE to learn how to use them.
Search#
The following example subclasses SearchKeywordInteraction()
to
implement search for docs.zyte.com:
import { SearchKeywordInteraction } from "smartbrowser-core-interactions/index.ts";
export default class DocsZyteComSearchKeywordInteraction extends SearchKeywordInteraction {
domains = ["docs.zyte.com"];
keywordCssSelector = "input.sidebar-search";
}
To try the example, use https://docs.zyte.com/
as target URL,
{"keyword": "foo"}
as parameters, and any geolocation.
Note
If you debug the example in real time from the Zyte IDE, for the browser script to work you must widen the browser view until the Zyte docs search box becomes visible.
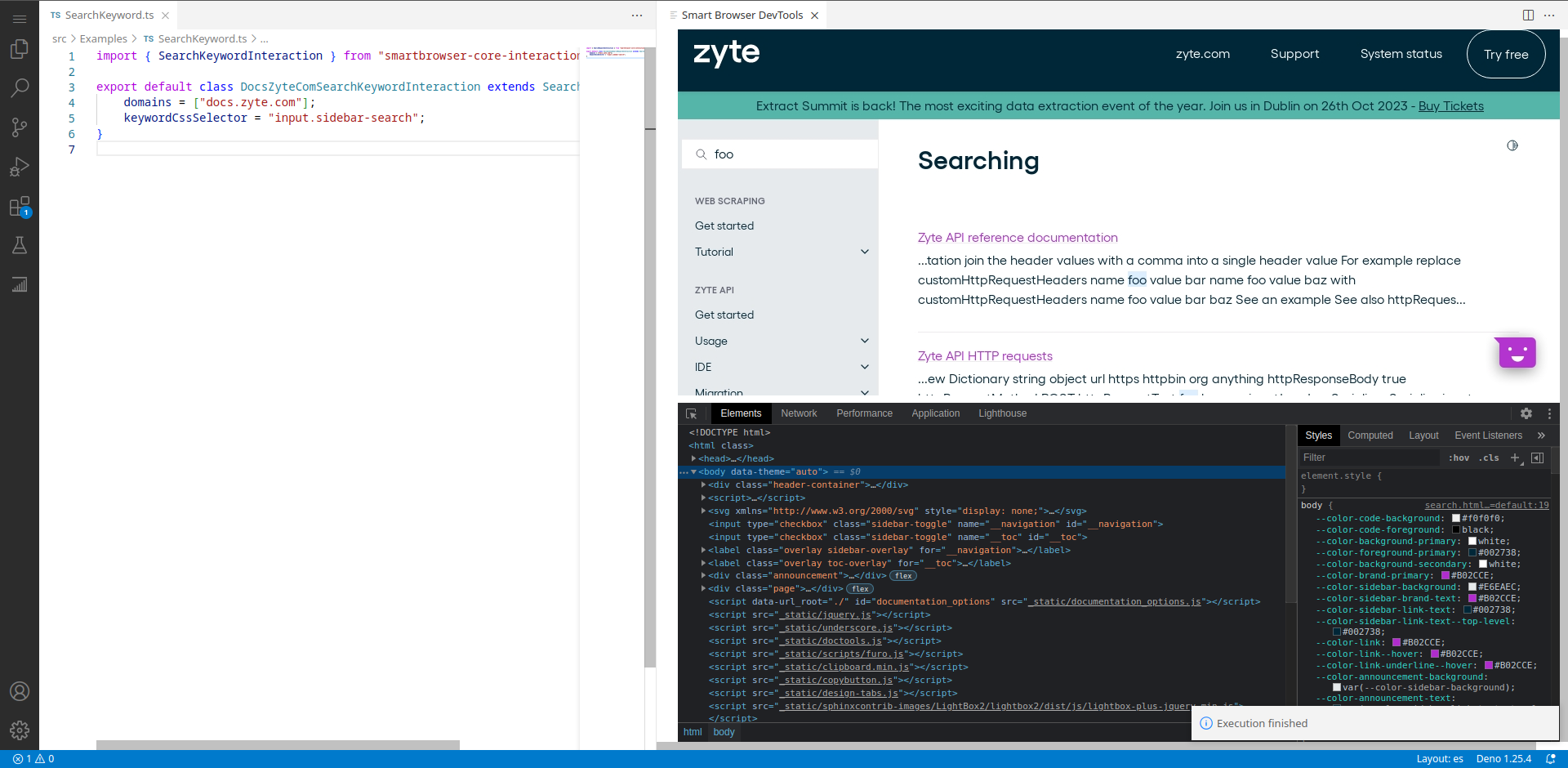
This is not a problem in a Zyte API requests, where the default viewport is wide enough.
Interactive form#
The following example fills and submits the content filtering form at quotes.toscrape.com/search.aspx:
import { BaseInteraction, Page } from "smartbrowser-core-interactions/index.ts";
interface Args {
author: string;
tag: string;
}
export default class QuotesToScrapeComSearchInteraction extends BaseInteraction {
domains = ["quotes.toscrape.com"];
async do(page: Page, args: Args): Promise<void> {
await page.select("#author", [args.author]);
await page.waitForSelector({type: "css", "value": "#tag option[value]", state: "attached"});
await page.select("#tag", [args.tag]);
await page.waitForTimeout(3);
await page.click('input[name="submit_button"]');
await page.waitForSelector(".quote");
}
}
To try the example, use https://quotes.toscrape.com/search.aspx
as target
URL, {"author": "Steve Martin", "tag": "humor"}
as parameters, and any
geolocation.
API call#
The following example shows how you can use JavaScript through the evaluate
action to send an API request from a browser and get
the API response in the resulting browserHtml:
import { BaseInteraction, Page } from "smartbrowser-core-interactions/index.ts";
interface Args {}
export default class QuotesToScrapeComAPICall extends BaseInteraction {
domains = ["quotes.toscrape.com"];
async do(page: Page, args: Args): Promise<void> {
const source = `
fetch("http://quotes.toscrape.com/api/quotes?page=1")
.then(response => response.json())
.then(data => {
document.write(JSON.stringify(data))
})
.catch(error => {
document.write(JSON.stringify({error}));
});
`;
await page.evaluate(source);
}
}
To try the example, use http://quotes.toscrape.com/scroll
as target
URL, {}
as parameters, and any geolocation.